Test coverage reports
Test coverage is an important indicator for the overall health of your project. Solid tests are a prerequisite for safe code refactoring and avoiding regressions and that is why we introduced coverage reporting as part of codebeat offering. You can integrate test coverage reports into your CI process for Ruby, JavaScript, TypeScript and Golang. Multiple language-specific reports can be uploaded for a single Codebeat project and will show in your project, as well as individual pull requests.
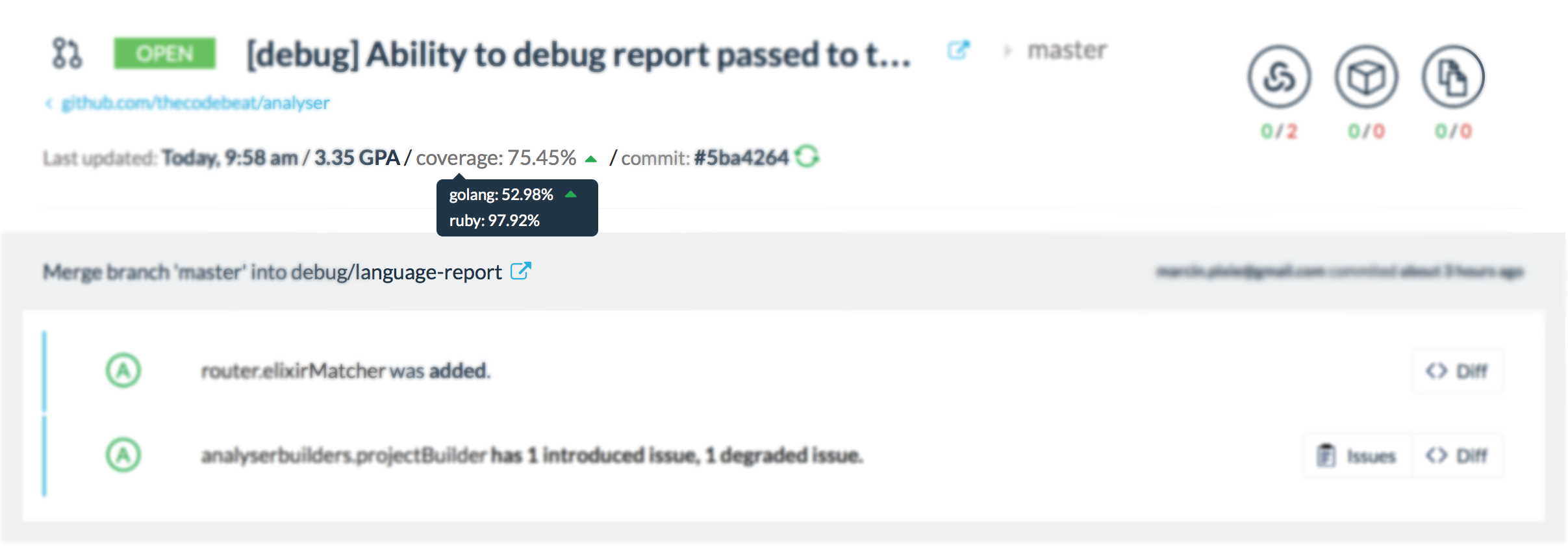
Multi-language code coverage indicator in one of Codebeat's own GitHub pull requests
Codebeat is compatible with two reporters: ruby-test-reporter and javascript-test-reporter, which are open-source libraries maintained by an industry leader - CodeClimate.
Please refer to individual setup guides listed below to get started:
Setting up for Ruby
Start by making sure your tests are reporting coverage files at the end of each run by adding the simplecov
gem to your Gemfile
and updating your dependencies. Also add codeclimate-test-reporter
gem for uploading reports to codebeat
group :test do
gem 'simplecov', require: false
gem 'codeclimate-test-reporter', require: false
end
Then, we need to ensure simplecov
is required and initialized before we run any test code. Add the following snippet at the very top of your spec/spec_helper.rb
or test/test_helper.rb
(depending on your test framework). You can read more in SimpleCov readme
require 'simplecov'
SimpleCov.start
The last step is to configure uploading test results by executing codeclimate-test-reporter
. You need to set two environment variables to indicate the address of Codebeat's API endpoint in CODECLIMATE_API_HOST
, as well as your project UUID set as CODECLIMATE_REPO_TOKEN
.
How do I find my Project UUID?
Project UUID resides in the Settings tab available from your project's home page.
Make sure to include the snippet below after specs are run in your CI environment, so that codeclimate-test-reporter
has results available to upload.
CODECLIMATE_API_HOST=https://codebeat.co/webhooks/code_coverage \
CODECLIMATE_REPO_TOKEN=<your-project-uuid> \
codeclimate-test-reporter
Setting up for JavaScript / TypeScript
Start by install the JavaScript flavour of codeclimate-test-reporter
. It can be added to your project environment or as a step in your CI routine.
npm install -g codeclimate-test-reporter
Generate test coverage files. Codebeat currently supports lcov
format, that can be generated by tools like Istanbul or karma-coverage.
// example coverage configuration for karma-runner
module.exports = function (config) {
var configuration = {
// ...
coverageReporter: {
type: 'lcovonly'
}
// ...
}
config.set(configuration);
}
Upload data to Codebeat after your tests are finished by passing the generated coverage file to codeclimate-test-reporter
. The example assumes it located in coverage/lcov/lcov.info
. Do not forget about setting CODECLIMATE_API_HOST
as per the example and CODECLIMATE_REPO_TOKEN
to your Project UUID, which can be obtained on the Settings page.
CODECLIMATE_API_HOST=https://codebeat.co/webhooks/code_coverage \
CODECLIMATE_REPO_TOKEN=<your-project-uuid> \
codeclimate-test-reporter < coverage/lcov/lcov.info
Setting up for Go
Golang is supported by the cover
format, which is built into the language toolchain. Run your tests with coverage output by appending the -coverprofile
flag to the test
command.
go test -v -coverprofile=coverage.out
Then you can use the JavaScript codeclimate-test-reporter
tool to upload the generated file to Codebeat.
npm install -g codeclimate-test-reporter
Set Codebeat's endpoint as CODECLIMATE_API_HOST
and your Project UUID as CODECLIMATE_REPO_TOKEN
and pass the generated coverage report as standard input for the command.
CODECLIMATE_API_HOST=https://codebeat.co/webhooks/code_coverage \
CODECLIMATE_REPO_TOKEN=<your-project-uuid> \
codeclimate-test-reporter < coverage.out
Testing multiple Go packages with coverage
Go's
cover
has an issue with testing multiple packages using the./...
operator. Combining-coverprofile
with./...
results in an error. You can try the temporary walkaround that we implemented for our CI pipeline.
Caveats
Please take note of the following constraints while implementing code coverage support for your project.
-
Coverage is asynchronous to Codebeat analysis. If by any chance you send more than one report for a given commit in a given language, Codebeat will override the old, per-language version.
-
Codebeat supports multiple per-language reports per project. Unfortunately, due to their asynchronous nature, your coverage status may become temporarily invalid, given a situation where coverage for one of the languages is still being processed.
-
Although we are working on it, Codebeat provides no integrations for code coverage notifications at the moment of writing this article.
Please contact our support in case of any issues or questions regarding this document or coverage setup in general.
Updated over 7 years ago